1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
//! **Integrator** is an abstract base class that defines the
//! **render()** method that must be provided by all integrators.
//!
//! - AOIntegrator
//! - BDPTIntegrator
//! - DirectLightingIntegrator
//! - MLTIntegrator
//! - PathIntegrator
//! - SPPMIntegrator
//! - VolPathIntegrator
//! - WhittedIntegrator
//!
//! ## Ambient Occlusion (AO)
//!
//! Ambient Occlusion is most often calculated by casting rays in
//! every direction from the surface. Rays which reach the background
//! or sky increase the brightness of the surface, whereas a ray which
//! hits any other object contributes no illumination. As a result,
//! points surrounded by a large amount of geometry are rendered dark,
//! whereas points with little geometry on the visible hemisphere
//! appear light.
//!
//! 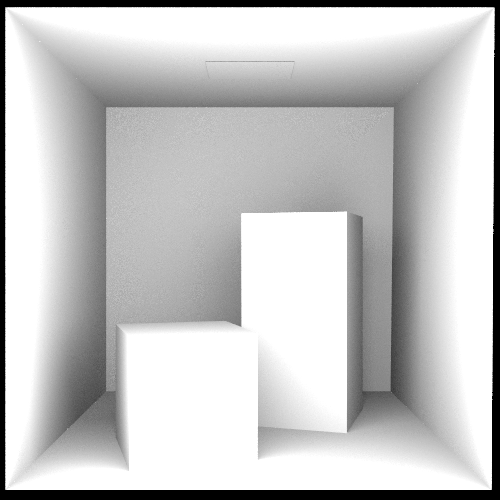
//!
//! ## Direct Lighting
//!
//! The **DirectLightingIntegrator** accounts only for direct lighting
//! — light that has traveled directly from a light source to the
//! point being shaded — and ignores indirect illumination from
//! objects that are not themselfes emissive, except for basic
//! specular reflection and transmission effects.
//!
//! 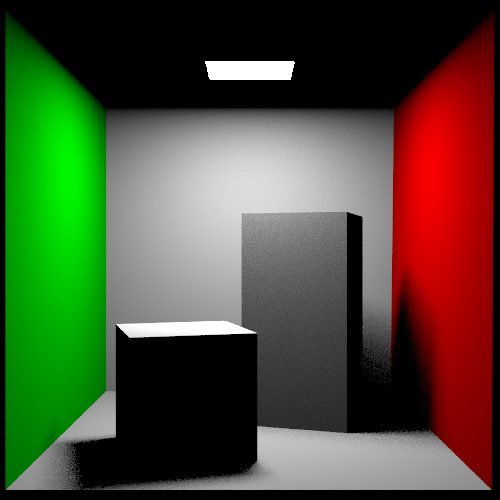
//!
//! ## Path Tracing
//!
//! Path tracing incrementally generates paths of scattering events
//! starting at the camera and ending at light sources in the scene.
//!
//! 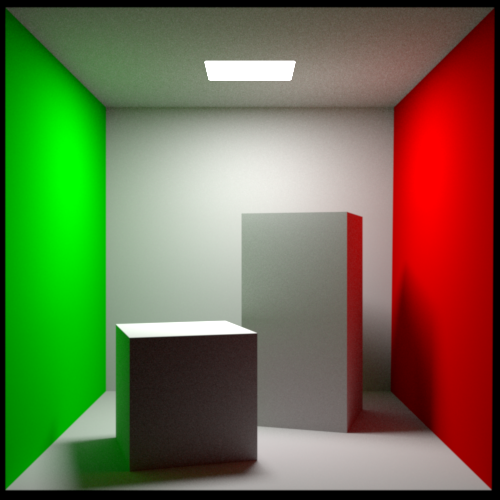
//!
//! ## Bidirectional Path Tracing (BDPT)
//!
//! Bidirectional path tracing is a generalization of the standard
//! pathtracing algorithm that can be much more efficient. It
//! constructs paths that start from the camera on one end, from the
//! light on the other end, and connects in the middle with a
//! visibility ray.
//!
//! 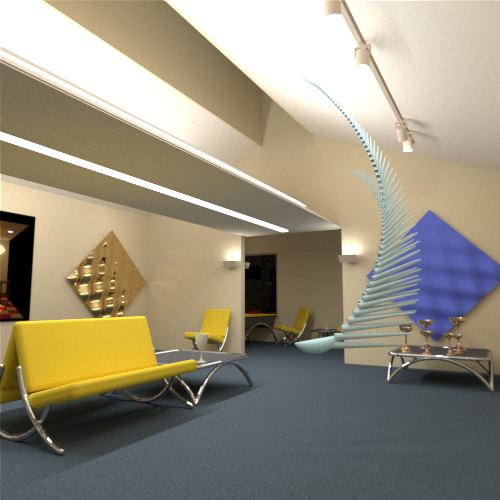
//!
//! ## Stochastic Progressive Photon Mapping (SPPM)
//!
//! A photon mapping integrator that uses particles to estimate
//! illumination by interpolating lighting contributions from
//! particles close to but not quite at the point being shaded.
//!
//! 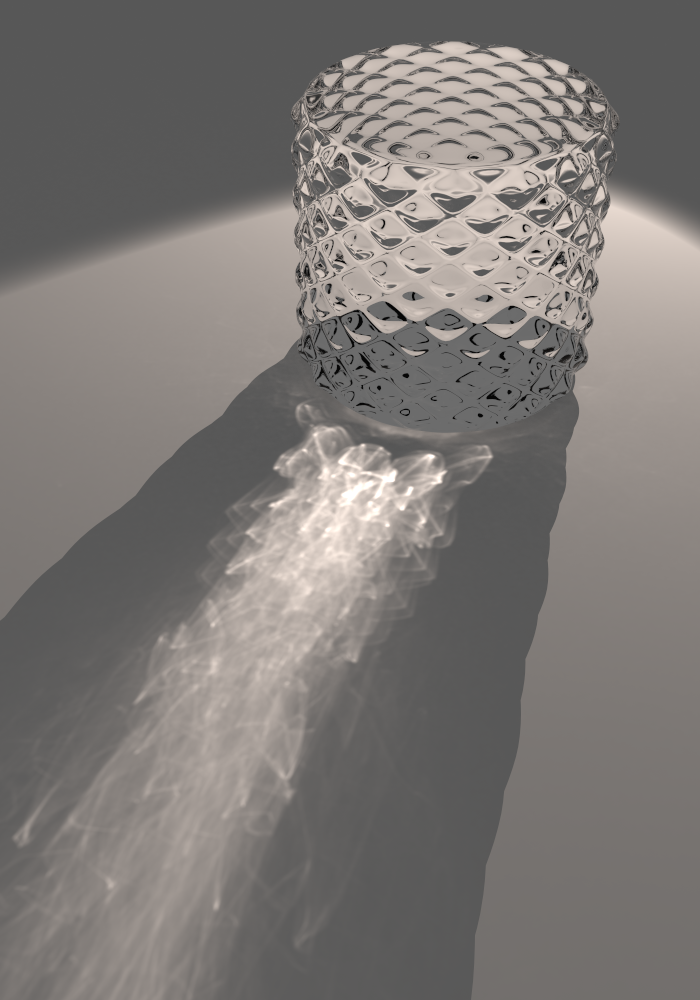
pub mod ao;
pub mod bdpt;
pub mod directlighting;
pub mod mlt;
pub mod path;
pub mod sppm;
pub mod volpath;
pub mod whitted;